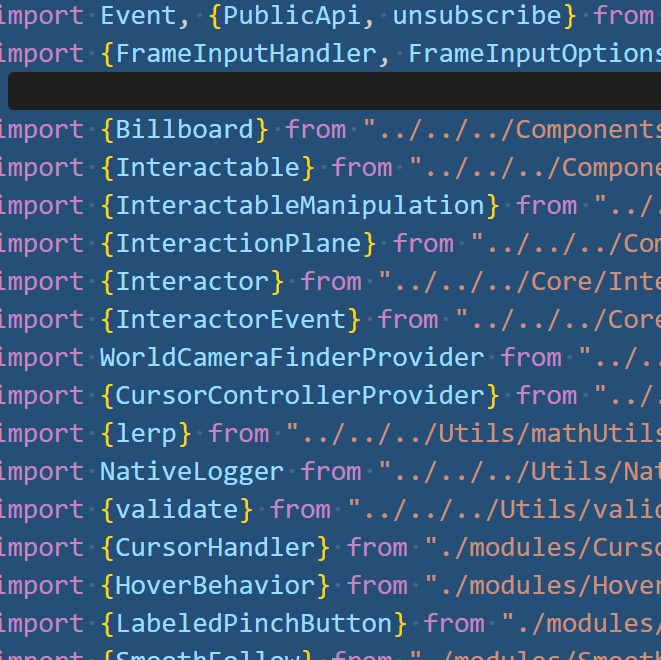
import animate, {AnimationManager, CancelSet} from "../../../Utils/animate" import Event, {PublicApi, unsubscribe} from "../../../Utils/Event" import {FrameInputHandler, FrameInputOptions} from "./modules/FrameInputHandler" import {Billboard} from "../../../Components/Interaction/Billboard/Billboard" import {Interactable} from "../../../Components/Interaction/Interactable/Interactable" import {InteractableManipulation} from "../../../Components/Interaction/InteractableManipulation/InteractableManipulation" import {InteractionPlane} from "../../../Components/Interaction/InteractionPlane/InteractionPlane" import {Interactor} from "../../../Core/Interactor/Interactor" import {InteractorEvent} from "../../../Core/Interactor/InteractorEvent" import WorldCameraFinderProvider from "../../../Providers/CameraProvider/WorldCameraFinderProvider" import {CursorControllerProvider} from "../../../Providers/CursorControllerProvider/CursorControllerProvider" import {lerp} from "../../../Utils/mathUtils" import NativeLogger from "../../../Utils/NativeLogger" import {validate} from "../../../Utils/validate" import {CursorHandler} from "./modules/CursorHandler" import {HoverBehavior} from "./modules/HoverBehavior" import {LabeledPinchButton} from "./modules/LabeledPinchButton" import {SmoothFollow} from "./modules/SmoothFollow" import {SnappableBehavior} from "./modules/SnappableBehavior" const log = new NativeLogger("ContainerFrame") export type InputState = { isHovered: boolean rawHovered: boolean isPinching: boolean position: vec3 drag: vec3 innerInteractableActive: boolean } export type ContainerFrameConfig = { target: SceneObject parent: SceneObject } const OPACITY_TWEEN_DURATION = 0.2 const SQUEEZE_TWEEN_DURATION = 0.4 const DEFAULT_BACKING_ALPHA = 1 const CAPTURE_BACKING_ALPHA = 0.1 /* * use forced depth for now * only 2d content */ const scaleZ = 1 /* * ratio for scaling between world space and margin space * testing different values here, leaving for reference during continued visdev * const scaleFactor = 90 * const scaleFactor = 70 */ const scaleFactor = 50 // frame depth factor const zScaleAdjuster = 15 const CURSOR_HIGHLIGHT_ANIMATION_DURATION = 0.15 /** * History of this magic number: * I added the frame mesh to the scene with a basic material * And overlayed it on a Unit Plane * I switched the camera to orthographic * And scaled up the Frame Mesh until it's edges just touched the edges of the unit plane * That was at scale 8.24 * So without any stretching, the mesh is 1 / 8.24 * 1 / 8.24 = 0.1213592233 * And since we set the base scale of the mesh to a constant and then stretch with vertex shader * I multiply that base scale by this number above, to get the size of the frame that isn't stretched * And subtract that from the amount we need to stretch * γ½(ο½ΠΒ΄)βββοΎ. * ο½₯ qοΎ, */ const magicScalar = 0.1213592233 /** * the base scale of the frame */ const scaleFactorVector = new vec3( scaleFactor + magicSc